Ray Tracer, C++
This is a basic, naive ray tracer with reflection and refraction. It actually performs fairly well despite the naive implementation. The 50 tiny spheres are placed randomly between the three larger spheres. At 600x450 resolution, it loads in about 45sec on my P4 3.0GHz HT computer. I will upload a sample of my source code in the future.
- ray tracer.zip (99.3 kb)
- Sample source coming soon!
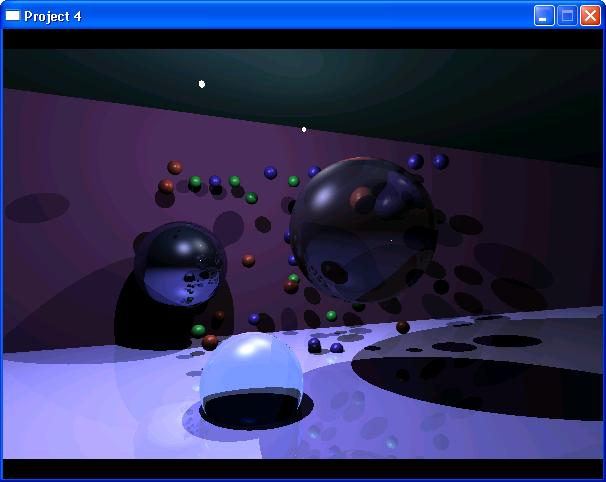
Shadows, C++ and OpenGL
Here I used shadow maps to do shadows. The boxes are drawn in random positions with tiny balls above them to better show shadows projecting on other objects. The light source is also movable.
- shadows.zip (98.8 kb)
- Source Code (17.2 kb)
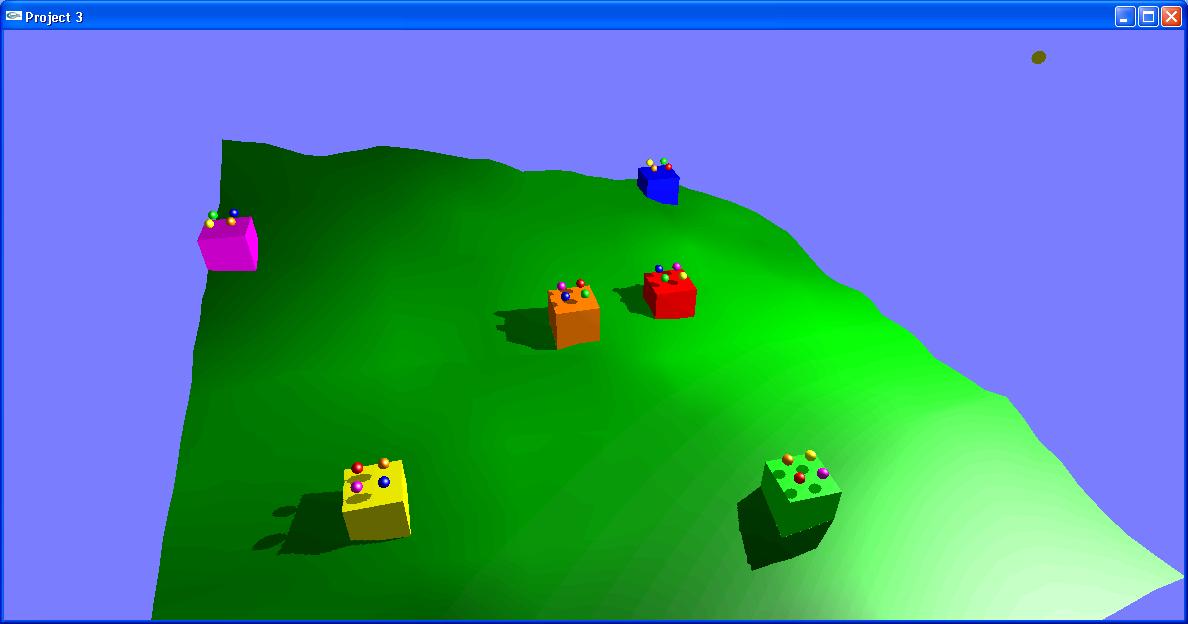
Airline Reservation System, Java
This is a Java prototype of an Airline Reservation System. It was a team-based project consisting of three members total. We used Eclipse, CVS, JUnit, and Ant for our implementation and testing. The program itself is big, but it is quite in its infant stage still.
- ARS.jar (347 kb)
- FileManager.java (18.7 kb)
- FlightSearch.java (5.49 kb)
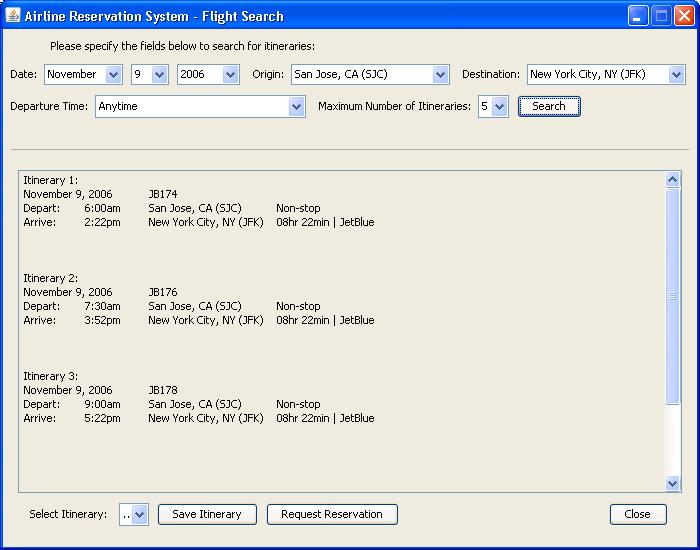
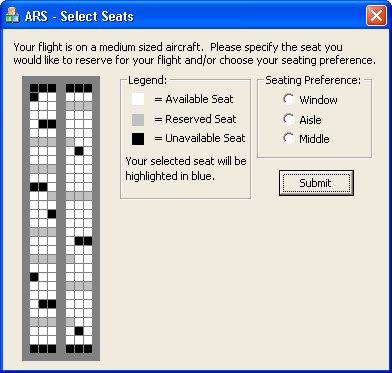
Ghost, ANSI C
Ghost is a word game in which players take turns adding letters to a growing word fragment, trying not to be the one to complete a valid word. The one that does loses that round of the game starts the new round. Each fragment must be the beginning of an actual word. The function takes a substring and a dictionary and prints out:
- the list of guaranteed win characters and their associated words
- the list of guaranteed lose characters and their words
- the list of keep-alive letters which may result in a win, but excluding guaranteed lose words if that letter is chosen.
A table of maps was implemented to store all the candidates that start with the input substring, as well as the three lists for the print-outs. I used a fairy simple parity-check algorithm to determine the guaranteed wins, guaranteed loses, and keep-alives.
- Executable (67.1 kb)
- Source Code (6.26 kb)
- Source + exe (121 kb)
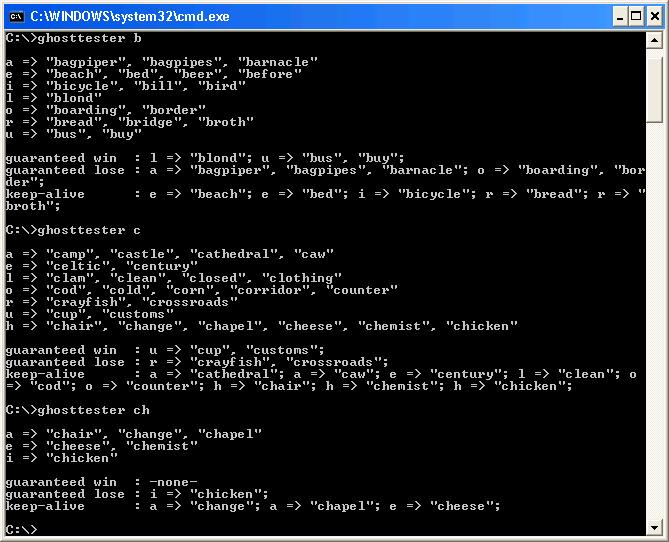
Tic-Tac-Toe, Java
This is a single player Tic-Tac-Toe game. The computer will never lose to the player in this Tic-Tac-Toe. The AI is implemented using a Game Tree. Even though there are easier, simpler ways of implementing a never-losing Tic-Tac-Toe algorithm, this way is much more flexible and can easily be adapted into any other non-complex game strategy.
- tictactoe.jar (6.82 kb)
- TTTPanel.java (11.0 kb)
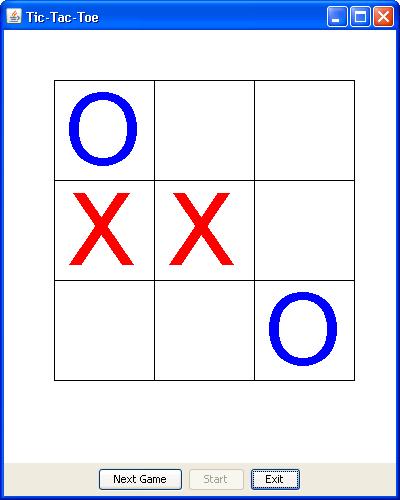
Maze, Java
This is a simple Maze creator and solver. Basically you click on walls to make them paths (and vice versa). If you click on Solve, it will attempt to find a solution with the path you have provided. The algorithm used is a simple recursive algorithm with fail-safe to prevent infinite recursion and also to prevent halts for when there is no solution. If I were to redo this program, I would add three things:
- A* or Dijkstra's Algorithm for solving the maze
- custom maze size
- click-hold-drag to turn walls into paths (and vice versa).
- maze.jar (6.49 kb)
- MazePanel.java (8.49 kb)
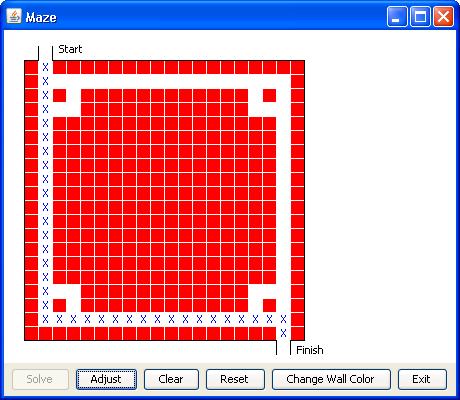
Procedural Textures, Cylindrical Billboarding, C++ and OpenGL
Here I implemented Perlin noise to create a procedural terrain texture and pasted that onto my terrain. Depending on the height, it will create a different color for grass, snow, and lakes. Then i used cylindrical billboarding to make the 2D texture trees always face the camera to make them seem 3D. The second screenshot has the billboarding turned off and you can see the effect very clearly. Because its cylindrical billboarding, going directly above a tree and looking down will still reveal the 2D nature of the textures. The second screenshot shows the billboarding turned off.
- billboarding.zip (379 kb)
- Source Code (16.9 kb)
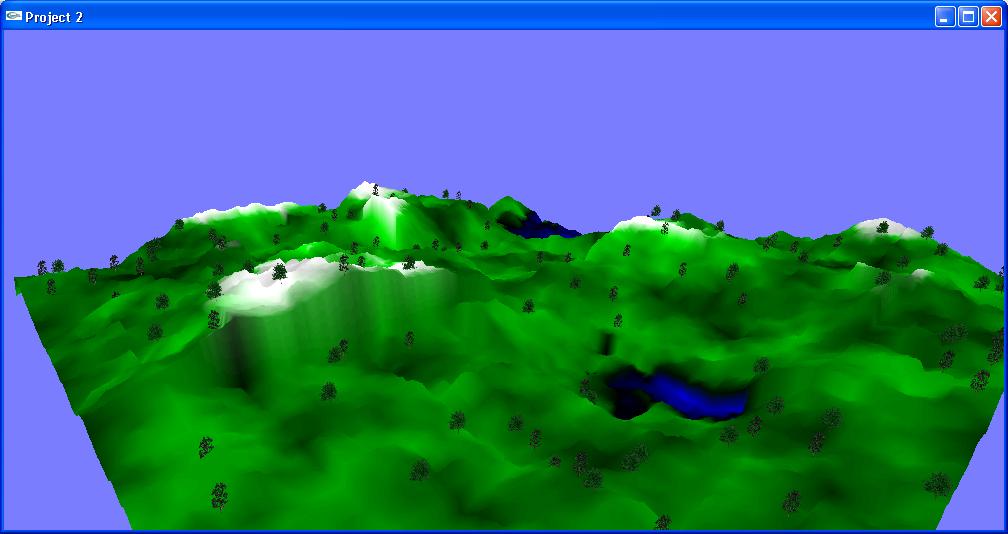
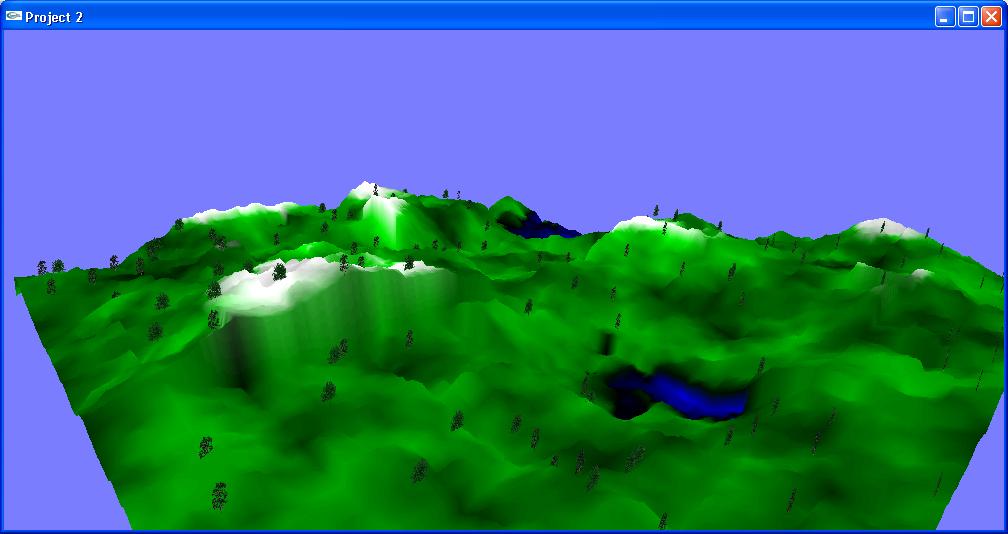